Advanced (JSON) Risk Configuration
REQUIRES 'RISKS' MODULE
Risk Input
The risk input defines all the factors which contribute to a risk, each factor can have none, one or several weights assigned to it. The user can select each weight from a predefined list of weights.
Risk Factor Configuration
interface IRiskConfig {
factors?:IRiskConfigFactor[], // a list of factors contributing to risk levels, see below
....
}
interface IRiskConfigFactor {
type:string, // an unique identification of the factor
label:string, // the label displayed in the UI, where user can enter some text, e.g. "Cause" or "Effect"
weights: IRiskConfigFactorWeight[] // an array of definitions describing how the factor contributes to the risk level, each weight is a a drop down of values
hideTextInput?:boolean, // default: false, set to trueif there should not be a text input field to specify details
readonly?:boolean, // default: false, can be set to true if user should not be able to change the field
inputType?:IRiskConfigFactorInputType, // default:text (a single line text input), can be used to overwrite the input as a drop down selection or a text area
options?: IRiskConfigFactorOption[], // if inputType is "select" this contains the values which can be selected
}
type IRiskConfigFactorInputType = "text"|"select"|"textarea";
interface IRiskConfigFactorOption {
value:string, // a unique identifier
label:string, // text to display
changes:IRiskConfigSelectChanges[] // for input select only. Allows to automatically change other inputs / weights when the select changes
}
interface IRiskConfigSelectChanges {
changesFactor?:string, // identifier (type) the factor to be modified OR
changesWeight?:string, // identifier (type) the weight to be modified
value:number // the new value to set
}
interface IRiskConfigFactorWeight {
type:string, // an identifier of the contributing factor
help:boolean, // if set true a help popover will explain the values
label:string, // the label displayed in the UI, where the user can select a value from the drop down, e.g. "Probability", "Severit"y or "Detectability"
readonly?:boolean, // default false, set to true, if an input field should not be changeable by the user
hidden?:boolean, // default false, set to true, if field should be hidden
values:IRiskConfigFactorWeightValue[] // an array of options of the drop down
}
interface IRiskConfigFactorWeightValue {
shortname:string,// name displayed in the UI, can omitted to only show the factor
help:string,// name displayed in tool tip help
factor:number //factor which goes into calculation of risk
}
Risk Assessment - before risk controls
See Risk Configuration Options
The method is defined by setting the property method to to either '*', '+' or 'lookup'. The property rbm can be set to hide or modify the text displayed in the UI or reports.
Definition Risk Assessment Method
interface IRiskConfig {
method:IRiskConfigMethod, // "+" or "*" whether to add or multiply the risk factors, "lookup" to get values from lookup table
...
rbm?:IRiskConfigRT,// allows to overwrite the default text used for Risk Before Mitigation fields.
}
type IRiskConfigMethod = "+"|"*"|"lookup";
interface IRiskConfigRT {
short:string, // text used in UI just before the field
long:string, // text used in tooltip in UI
report:string, // text used in report
hidden?:boolean // default:false, set to true if this should not be displayed/reported
}
Risk assessment - Method 1
Method one is defined by the setting method to '*' or '+'. If defined the following properties must be defined as well, in order to define the classification based on the sum / product.
Sum or Product of Weights
interface IRiskConfig {
...
maxGreen?:number, // the maximum risk level value which will be shown in green (low risk)
maxYellow?:number, // the maximum risk level value which will be shown in yellow (moderate risk), all above will be red (high risk)
...
}
Risk assessment - Method 2
Method two is defined by the setting method to 'lookup'. If defined the following properties must be defined as well, in order to define the classification based on the sum / product.
Lookup of Weights
interface IRiskConfig {
...
charts?:IRiskConfigZone[], // colors and text per risk class
rpns?:IRiskConfigRPN[], // lookup table to determine for risk priority numbers based on weights
...
}
interface IRiskConfigZone {
zone?:string, // unqiue ID
foreground?:string, // foreground color in the user interface
background?:string, // background color in the user interface
textColor?:string, // text color in reports
label?:string // text to be displayed
}
interface IRiskConfigRPN { // e.g. { "probability":5, "severity":4, "zone":"LOW", "text":"5 x 4 = 20" }
zone:string, // ID of zone
text:string, // for below combination of numbers: the resulting zone(char) and text
[key:string]:string|number // properties for each weight defining the lookup value for this lookup entry
}
Risk Controls
Risk controls are defined by selecting or creating items from or more categories (usually these are considered as design input).
The section name "Risk controls" in the UI and in the printed report can be modified in the advanced settings of the Risk configuration. For example, if you want to change it to "My risk controls", just adapt the JSON below the "riskconfig" part in the advanced settings:
You can also add "control":"one control is" - to used as prefix for each risk control when printing risk items. If set, when printing a report containing risk, each risk control would be prefixed by "one control".
Video:
See the FAQ on "How to add a category as Risk control?".
Risk Reduction Settings
interface IRiskConfig {
...
mitigationTypes?:IRiskConfigMitgationType[], // a list of categories which can be used as risk controls, see below. If there are no mitigations the RBM section will not be shown.
reductions?:IRiskConfigReduction[], // a list describing how risk levels can be reduced by risk controls, see below.
postReduction?:IRiskPostReduction // option for user selection after risk controls
...
}
Method 1 - Reduction per risk control
For this method, the reductions property must be configured. It is a an array of IRiskConfigReduction options.
Risk Reduction per Risk Control
interface IRiskConfigReduction {
name:string,// a unqiue id of the reduction factor
options:IRiskConfigReductionOptions[] // an array of reductions factors describing how the risk level can be reduced. These show up as drop down of values:
}
interface IRiskConfigReductionOptions {
shortname:string, // the string shown in drop down
by: number, // the amount by which a factor of the risk is changed (so negative values reduce the risk level)
changes:string, // defines which weight is reduced.
}
Method 2 - Global risk reduction
If the user has selected at least one risk control, this option allows the to user modify one or more of the weights defined as risk input. The weights which can be changed must be defined with all options which can be selected. These can be the same weight definitions as in the risk specifications itself.
Global Risk Reduction
interface IRiskPostReduction {
weights:IRiskConfigFactorWeight[]
}
Risk Assessment After Risk Controls
The method used is the same as the risk assessment before risk controls. The property ram can be set to hide or modify the text displayed in the UI or reports.
Definition Risk Assessment Method
interface IRiskConfig {
...
ram?:IRiskConfigRT,// allows to overwrite the default text used for Risk After Mitigation fields.
}
interface IRiskConfigRT {
short:string, // text used in UI just before the field
long:string, // text used in tooltip in UI
report:string, // text used in report
hidden?:boolean // default:false, set to true if this should not be displayed/reported
}
Updates after configuration changes
Note: If you already have some risks in the projects. you can still change the configuration, but you will to manually review and save eave each risk afterwards, since the changes might have a regulatory impact.
If you are sure that nothing changed in that regards, e.g. if you only fixed a spelling mistake, you can also automate this:
When you save the changed configuration you will be offered to re-index the database, this is a feature you can also enable in the Advanced Features.
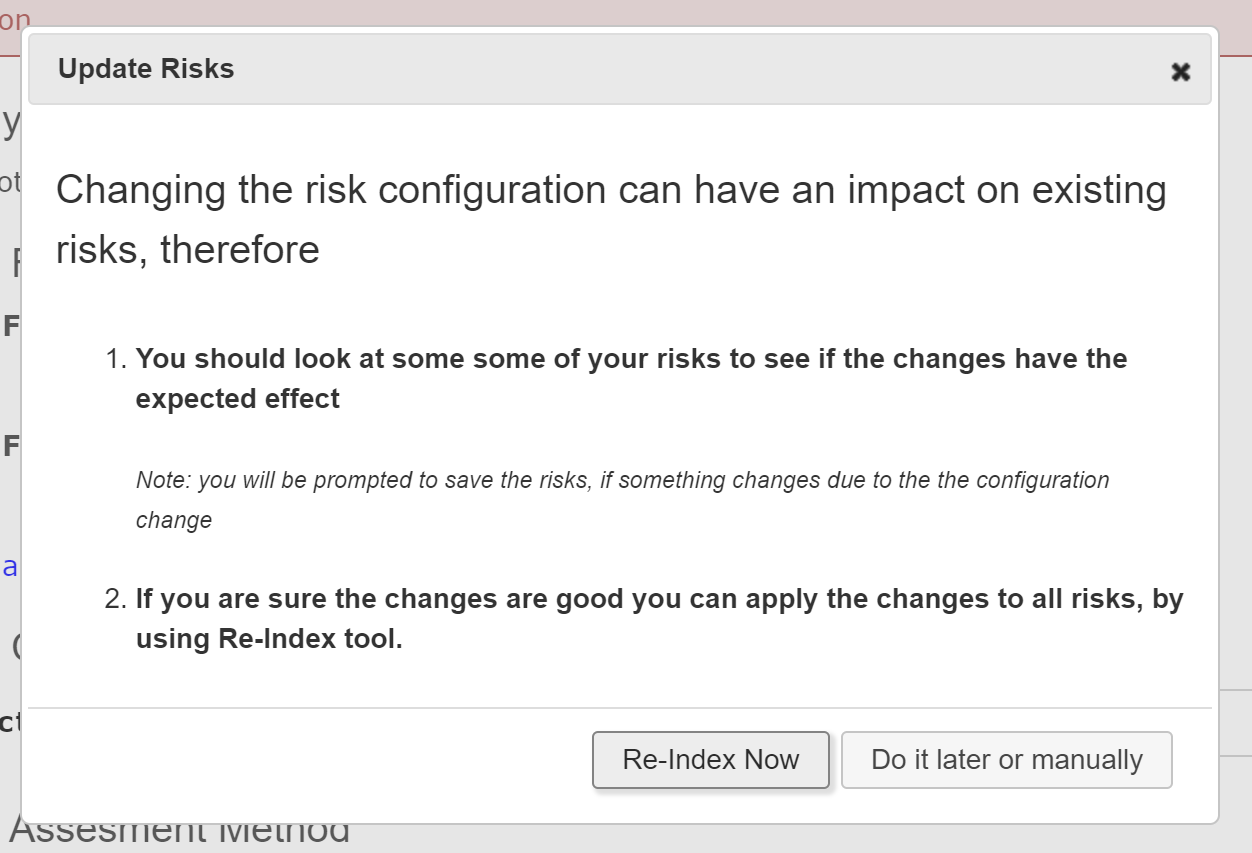
Examples